Prox
Forum adept
- Joined
- Sep 9, 2020
- Messages
- 384
- Reaction score
- 31
Whether you pronounce it "reg x", "rejects", "reggix", or even "riggex", RegEx, or a regular expression, is a helpful tool for most situations.
Enabling RegEx
To start using RegEx, you need to know how to enable it, because it doesn't start out enabled.
RegEx is available in three code actions, If Variable: Text Matches, Set Variable: Remove Text, and Set Variable: Replace Text. Each of these have the Regular Expressions tag, set to Disabled by default. To enable RegEx, click this tag to set it to Enabled.
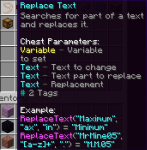
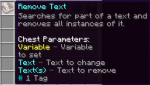
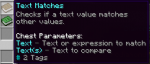
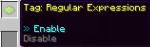
Learning RegEx
Before you jump in, you should also learn how to use it. You could look up "RegEx Tutorial", but there's one tiny problem: there are multiple kinds of RegEx. DiamondFire specifically uses Java RegEx, since Minecraft is built on Java. When you're learning RegEx, It's also a good idea to write RegEx along the way. There are many online RegEx engines you can do this with, but I like to use this one, since it's without a doubt Java RegEx. Here are some useful rules:
There is a good chance that if you use
Special note: With Replace Text, if you used capture groups in your RegEx, and also used character sets, quantifiers, or other "metacharacters" inside the group, but want to use what was matched inside the group in your replacement text, you can use $X in the replacement, where X is the number of the group you want the match value of.
Use cases include:
Here are some examples of RegEx that I've actually used.
Just note that RegEx is not always necessary, it's just useful for when you want to save on codespace, and makes code legibility terrible, especially for anyone who doesn't understand it very well. I would not recommend asking for help on fixing your RegEx with
Enabling RegEx
To start using RegEx, you need to know how to enable it, because it doesn't start out enabled.
RegEx is available in three code actions, If Variable: Text Matches, Set Variable: Remove Text, and Set Variable: Replace Text. Each of these have the Regular Expressions tag, set to Disabled by default. To enable RegEx, click this tag to set it to Enabled.
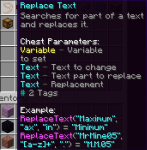
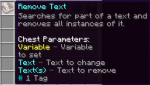
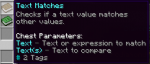
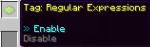
Learning RegEx
Before you jump in, you should also learn how to use it. You could look up "RegEx Tutorial", but there's one tiny problem: there are multiple kinds of RegEx. DiamondFire specifically uses Java RegEx, since Minecraft is built on Java. When you're learning RegEx, It's also a good idea to write RegEx along the way. There are many online RegEx engines you can do this with, but I like to use this one, since it's without a doubt Java RegEx. Here are some useful rules:
- If you want to match "win" or "won", you can set your RegEx to "w[io]n". Any character between the brackets will be matched.
- If you want to match anything, you can use a period ( . ), as it will match quite literally any character. This is a metacharacter.
- If you want to match multiple of a character, put + or * after it. + makes sure that there's at least one, where * still matches if there isn't any of it. These are called quantifiers.
- If you want a character to be optional, but not match multiple like * would, put ? after it. ? matches if there is zero or one of the preceding character.
- You can apply quantifiers to a group of characters by surrounding the group with parenthesis, like (this). These are called capture groups
- You can also use quantifiers on character sets, such as the "w[io]n" example above. (w[io]+n would also match woon, wiin, even wion)
- You can match a character a certain number of times by putting {x} after it. Replace x with the number of times to match.
- You can match a character a range of times by putting {x,y} after it. x is the minimum, y is the maximum. You can exclude x if it's zero, and exclude y if you want just a minimum So, for a minimum of three times, use {3,}. This is also a quantifier.
- \s, \d, and \w match any and all spaces, numbers, and letters, respectively. \S, \D, and \W match anything but. These are metacharacters.
- If you want to use a character such as ., {, }, [, ], $, ^, !, ?, or \, you should put a \ before it.
There is a good chance that if you use
/txt
to get your RegEx text, your RegEx may be faulty due to square brackets being interpreted as a way to get multiple texts at once (/txt w[i,o]n, for example, would give win
AND won
texts). To combat this, get an insignificant text, such as /txt a
and then edit that text to be your RegEx.Special note: With Replace Text, if you used capture groups in your RegEx, and also used character sets, quantifiers, or other "metacharacters" inside the group, but want to use what was matched inside the group in your replacement text, you can use $X in the replacement, where X is the number of the group you want the match value of.
Use cases include:
- Matching certain patterns in text, such as the patterns for different variable types in those variables'
varitem
item tag. - Extracting specific data from a text, such as the X, Y, Z, Pitch, and Yaw values from a location's
varitem
tag. - Testing user input to make sure it follows (or does not follow) a pattern, such as a hex code (#FFFFFF) or its & code equivalent (&x&F&F&F&F&F&F)
Here are some examples of RegEx that I've actually used.
\{"id":"txt","data":\{"name":".*"\}\}
will match thevaritem
tag of a text variable.\{"id":"loc","data":\{"isBlock":false,"loc":\{"x":(-?\d+\.\d+),"y":(-?\d+\.\d+),"z":(-?\d+\.\d+),"pitch":(-?\d+\.\d+),"yaw":(-?\d+\.\d+)\}\}\}
will let you get the x, y, z, pitch, and yaw values from a location variable'svaritem
tag. (These two are useful for when you have variables in chests or barrels in the plot area)#[\da-fA-F]{6}
will match hex codes such as #f5dE72
Just note that RegEx is not always necessary, it's just useful for when you want to save on codespace, and makes code legibility terrible, especially for anyone who doesn't understand it very well. I would not recommend asking for help on fixing your RegEx with
/support request
, because there is a chance that your support member will not understand how to use RegEx. The same problem probably also lies within /support question
, because no online support may understand either, but if you can't solve it yourself, it's your best bet. If no one understands RegEx, you could either test aggressively with the site I mentioned above, or ask a Developer such as Owen1212055 on Discord. (Note: Discord is meant for users at least 13 years old, and a developer may be busy at some point.)
Last edited: